For Axon.ivy 6.1 or younger!
Yes this is quite simple. Just annotate a java service class with JAX-RS annotations to define the interface of your public service.
/**
* Provides the person REST resource
* on the path /ivy/api/myApplicationName/person
*/
@Path("{applicationName}/person")
public class CustomProjectResource {
@GET
@Produces(MediaType.APPLICATION_JSON)
public Person get() {
Person p = new Person();
p.setFirstname("Renato");
p.setLastname("Stalder");
return p;
}
}
The ConnectivityDemos project contains many sample services that demonstrates a REST service definition via JAX-RS annotations. See for instance the com.axonivy.connectivity.rest.provider.PersonService
.
See also the 3rd Party Integration documentation: http://developer.axonivy.com/doc/latest/DesignerGuideHtml/ivy.integration.html#ivy-integration-rest
With 6.0 or older REST API definition is not supported. But you can anyway provide a simple HTTP service that can be used by REST clients by applying the following solutions:
PROVIDE DATA (Axon.ivy 6.0 and older)
If you just want to send business-data (java objects) to a REST client the solution is quit simple. :
- Create a new RequestStart event as entry URL for the REST request. The start event should not have any input parameters or result configured.
- Load the data to return (either in plain java or with a process flow)
- Let the process flow end on a Script activity. This activity is responsible to end the Request and write the serialized JSON directly to the HTTP response. See
ch.ivyteam.q.and.a.RestRequest.sendAsResponse(String)
in the sample project
See simpleRestProvider_51-v2.iar customers/get.ivp for a sample .
CONSUME AND PROVIDE DATA (Axon.ivy 6.0 and older)
Solution if you need to get JSON parameters from the client as input, process them and return a result in JSON format.
- Create a new RequestStart event as entry URL for the REST request. The start event must have a single String parameter which will receive the serialized JSON object(s) from the REST client.
- De-serialize the input parameter within a Script activity. Use Gson or a similar library for the conversion from JSON to java. Map the de-serialized java object to the process data.
- Load the data to return (either in plain java or with a process flow)
- Let the process flow end on a Script activity. This activity is responsible to end the Request and write the serialized JSON directly to the HTTP response. See
ch.ivyteam.q.and.a.RestRequest.sendAsResponse(String)
in the sample project
See simpleRestProvider_51-v2.iar customers/filter.ivp for a sample. For quick review open the java class ch.ivyteam.q.and.a.TestRestMethods, open the context menu of the editor, choose: run as, junit test
Sample
-
Process
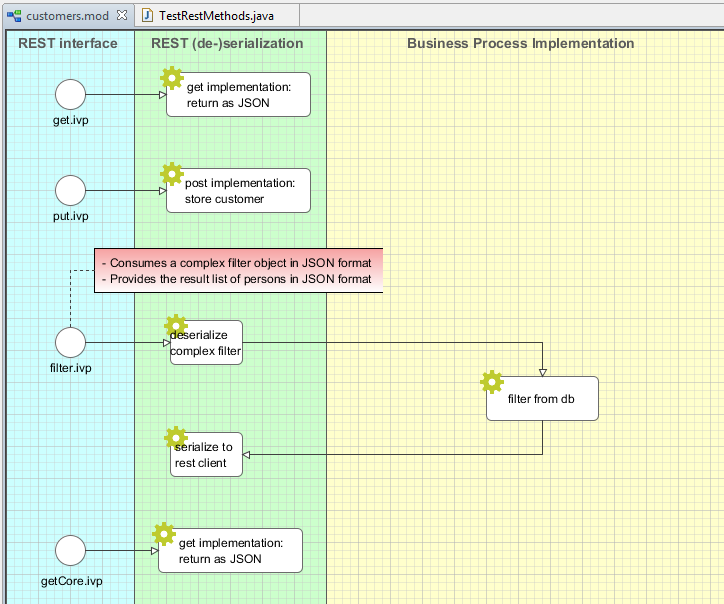
-
Rest Request helper
package ch.ivyteam.q.and.a;
import java.io.IOException;
import java.io.Writer;
import javax.servlet.http.HttpServletRequest;
import ch.ivyteam.ivy.environment.Ivy;
import ch.ivyteam.ivy.request.IHttpRequest;
import ch.ivyteam.ivy.request.IHttpResponse;
import ch.ivyteam.ivy.request.IRequest;
import ch.ivyteam.ivy.request.IResponse;
/*
* Rest request simplifies access to HTTP request/response.
* @author Reguel Wermelinger
/
public class RestRequest {
/**
* Ends the request and writes given response content manually. Should be called from a Script activity with no outgoing flow.
* @param json
*/
public static void sendAsResponse(String json){
try {
getHttpResponseWriter().append(json);
} catch (IOException e) {
Ivy.log().error("Failed to send json response.", e);
}
}
public static Writer getHttpResponseWriter() {
IResponse response = Ivy.response();
if (!(response instanceof IHttpResponse)) {
throw new RuntimeException(
"Response must be an HTTP response, but was " + response);
}
IHttpResponse httpResponse = (IHttpResponse) response;
try {
return httpResponse.getHttpServletResponse().getWriter();
} catch (IOException ex) {
throw new RuntimeException("Could not get HTTP response writer.", ex);
}
}
public static String getHttpMethod() {
return getHttpServletRequest().getMethod();
}
public static HttpServletRequest getHttpServletRequest() {
IRequest rootRequest = Ivy.request().getRootRequest();
if (!(rootRequest instanceof IHttpRequest)) {
throw new RuntimeException(
"request must be an HTTP request, but was " + rootRequest);
}
IHttpRequest httpRequest = (IHttpRequest) rootRequest;
HttpServletRequest httpServletRequest = httpRequest.getHttpServletRequest();
return httpServletRequest;
}
}
answered
31.08.2015 at 11:30
Reguel Werme... ♦♦
9.4k●3●19●58
accept rate:
70%