Ivy Version 7.0.2 and later
The REST Client respects the well-known ivy ssl settings. Enable your custom truststore and import the self signed certificat:
https://developer.axonivy.com/doc/latest/DesignerGuideHtml/ivy.introduction.html#ivy-introduction-preferences-sslclient
Ivy Version 7.0.1 and before
You have multiple options:
- Put the certificate into the engines trustStore
- Use a custom connector
Put the certificate into the engines trustStore
- import the certificate into the trusstore of the engine/designer under
configuration/truststore.jks
. I recommend to use something like the KeyStore explorer
to do this. 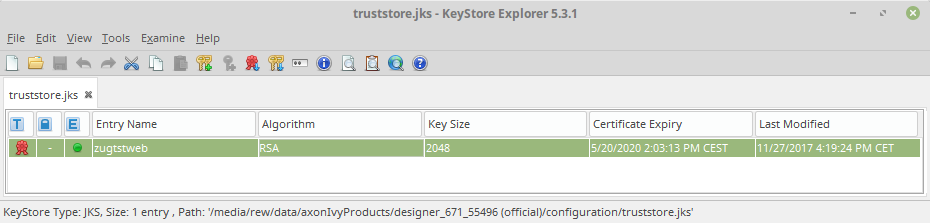
- activate the keystore globally for the JVM by setting system properties. You could do this either in a script/java call before the REST request is executed or in the
Axon.ivy Designer.ini
respectively the *.ilc
file of the engine.
.
javax.net.ssl.trustStore=configuration/truststore.jks
javax.net.ssl.trustStorePassword=changeit
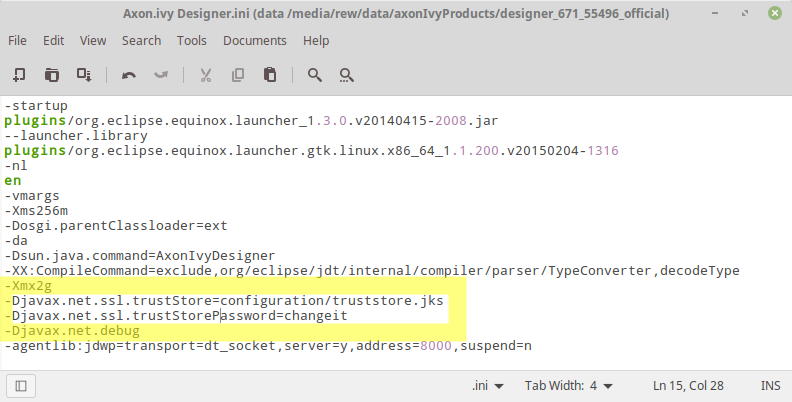
Use a custom connector which allows unsecure hosts:
Test with Axon.ivy 6.7.1. Currently this is a painful workaround.
- add the jersey-apache-connector.jar to the
/dropins
directory of your product
- copy the jersey-apache-connector.jar plus the jersey-commons.jar and the jersey-guava.jar into your project as well. And add them to the classpath.
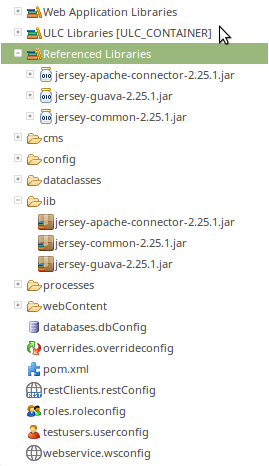
- enable the apache-connector-provider via SPI.
- -- Create a file: /src/META-INF/services/org.glassfish.jersey.client.spi.ConnectorProvider
- -- In it paste the full qualified name of the connector:
org.glassfish.jersey.apache.connector.ApacheConnectorProvider
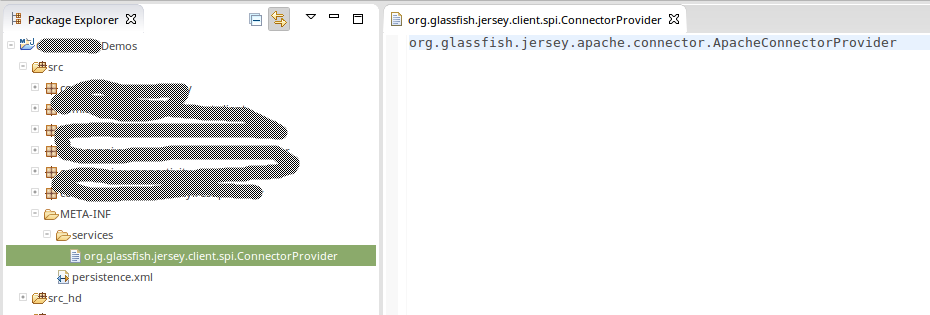
- add a java class that configures the apache-connector to allow any certificate by any host (see below)
- use
CustomRest.unsecureClient("myRestSErviceName")
as entry point for your REST requests. package com.axonivy.connectivity;
import java.security.SecureRandom;
import java.security.cert.CertificateException;
import java.security.cert.X509Certificate;
import javax.net.ssl.HostnameVerifier;
import javax.net.ssl.SSLContext;
import javax.net.ssl.SSLSession;
import javax.net.ssl.TrustManager;
import javax.net.ssl.X509TrustManager;
import javax.ws.rs.client.WebTarget;
import org.apache.http.config.Registry;
import org.apache.http.config.RegistryBuilder;
import org.apache.http.conn.HttpClientConnectionManager;
import org.apache.http.conn.socket.ConnectionSocketFactory;
import org.apache.http.conn.socket.PlainConnectionSocketFactory;
import org.apache.http.conn.ssl.SSLConnectionSocketFactory;
import org.apache.http.impl.conn.BasicHttpClientConnectionManager;
import org.glassfish.jersey.apache.connector.ApacheConnectorProvider;
import ch.ivyteam.ivy.environment.Ivy;
public class CustomRest {
public static WebTarget unsecureClient(String serviceName)
{
Thread th = Thread.currentThread();
ClassLoader oldCtl = th.getContextClassLoader();
try
{
th.setContextClassLoader(ApacheConnectorProvider.class.getClassLoader());
HttpClientConnectionManager conMan = new BasicHttpClientConnectionManager(getConnectionRegistry(), null, null, null);
return Ivy.rest().client(serviceName)
.property("jersey.config.apache.client.connectionManager", conMan);
}
finally
{
th.setContextClassLoader(oldCtl);
}
}
private static Registry<ConnectionSocketFactory> getConnectionRegistry() {
SSLContext ctxt = createTrustAllContext();
HostnameVerifier verifyAllHosts = new HostnameVerifier() {
@Override
public boolean verify(String hostname, SSLSession session) {
return true;
}
};
SSLConnectionSocketFactory factory = new org.apache.http.conn.ssl.SSLConnectionSocketFactory(ctxt, verifyAllHosts);
return RegistryBuilder.<ConnectionSocketFactory>create()
.register("http", PlainConnectionSocketFactory.getSocketFactory())
.register("https", factory)
.build();
}
private static SSLContext createTrustAllContext() {
TrustManager[] certs = new TrustManager[]{new X509TrustManager() {
@Override
public X509Certificate[] getAcceptedIssuers() {
return null;
}
@Override
public void checkServerTrusted(X509Certificate[] chain, String authType) throws CertificateException {
}
@Override
public void checkClientTrusted(X509Certificate[] chain, String authType) throws CertificateException {
}
}};
SSLContext ctx = null;
try {
ctx = SSLContext.getInstance("TLS");
ctx.init(null, certs, new SecureRandom());
} catch (java.security.GeneralSecurityException e) {
throw new RuntimeException("failed to setup insecure ssl context", e);
}
return ctx;
}
}
answered
29.08.2017 at 07:56
Reguel Werme... ♦♦
9.4k●3●19●58
accept rate:
70%